**体育赛事直播系统的炫彩弹幕直播间界面,“东莞梦幻网络科技”的源码技术实现方案,包括前端(Vue.js)、后端(ThinkPHP)、安卓(Java)和iOS(Objective-C)的实现代码。**
## 系统架构设计
**技术栈**
后端: PHP (ThinkPHP 6.x)
数据库: MySQL + Redis
前端: Vue.js 3 + Element Plus
移动端:
Android: Java + Retrofit
iOS: Objective-C + AFNetworking
实时通信: WebSocket (Swoole)
## 后端实现 (ThinkPHP)
**数据库设计**
```objectivec
// 直播表
Schema::create('live_streams', function (Blueprint $table) {
$table->id();
$table->string('title'); // 直播标题
$table->integer('sport_type'); // 运动类型
$table->string('home_team'); // 主队
$table->string('away_team'); // 客队
$table->datetime('start_time'); // 开始时间
$table->string('stream_url'); // 直播流地址
$table->integer('viewer_count')->default(0); // 观看人数
$table->integer('status')->default(0); // 状态 0-未开始 1-直播中 2-已结束
$table->timestamps();
});
// 弹幕表
Schema::create('barrages', function (Blueprint $table) {
$table->id();
$table->integer('live_id'); // 直播ID
$table->integer('user_id'); // 用户ID
$table->string('content'); // 弹幕内容
$table->string('color')->default('#FFFFFF'); // 颜色
$table->integer('position')->default(0); // 位置
$table->timestamps();
});
```
**控制器代码**
```objectivec
namespace app\controller;
use app\BaseController;
use think\facade\Db;
use think\facade\Request;
class LiveController extends BaseController
{
// 获取直播列表
public function getLiveList()
{
$page = Request::param('page', 1);
$size = Request::param('size', 10);
$list = Db::name('live_streams')
->where('status', '>=', 0)
->order('start_time', 'desc')
->paginate(['page' => $page, 'list_rows' => $size]);
return json([
'code' => 200,
'data' => $list->items(),
'total' => $list->total()
]);
}
// 获取直播详情
public function getLiveDetail($id)
{
$live = Db::name('live_streams')->find($id);
if (!$live) {
return json(['code' => 404, 'msg' => '直播不存在']);
}
return json([
'code' => 200,
'data' => $live
]);
}
// 发送弹幕
public function sendBarrage()
{
$data = Request::only(['live_id', 'content', 'color', 'position']);
$data['user_id'] = Request::middleware('user_id');
$id = Db::name('barrages')->insertGetId($data);
// 通过WebSocket广播弹幕
$this->app->swoole->pushToAll(json_encode([
'type' => 'barrage',
'data' => array_merge($data, ['id' => $id])
]));
return json(['code' => 200, 'msg' => '发送成功']);
}
// 获取弹幕列表
public function getBarrages($live_id)
{
$list = Db::name('barrages')
->where('live_id', $live_id)
->order('create_time', 'desc')
->limit(100)
->select();
return json([
'code' => 200,
'data' => $list
]);
}
}
```
## Web前端实现 (Vue.js)
**页面结构**
```objectivec
```
**Vue组件逻辑**
```objectivec
```
**样式部分**
```objectivec
```
## 移动端实现
**Android实现 (Java)**
```objectivec
// LiveActivity.java
public class LiveActivity extends AppCompatActivity {
private VideoView videoView;
private RecyclerView barrageRecyclerView;
private EditText barrageEdit;
private Button sendButton;
private LiveViewModel viewModel;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_live);
// 初始化视图
videoView = findViewById(R.id.video_view);
barrageRecyclerView = findViewById(R.id.barrage_recycler);
barrageEdit = findViewById(R.id.barrage_edit);
sendButton = findViewById(R.id.send_button);
// 设置布局管理器
barrageRecyclerView.setLayoutManager(new LinearLayoutManager(this));
// 初始化ViewModel
viewModel = new ViewModelProvider(this).get(LiveViewModel.class);
// 获取直播ID
String liveId = getIntent().getStringExtra("live_id");
// 观察数据变化
viewModel.getLiveData().observe(this, live -> {
// 更新UI
videoView.setVideoURI(Uri.parse(live.getStreamUrl()));
videoView.start();
});
viewModel.getBarrages().observe(this, barrages -> {
// 更新弹幕列表
BarrageAdapter adapter = new BarrageAdapter(barrages);
barrageRecyclerView.setAdapter(adapter);
});
// 发送弹幕
sendButton.setOnClickListener(v -> {
String content = barrageEdit.getText().toString();
if (!TextUtils.isEmpty(content)) {
viewModel.sendBarrage(liveId, content);
barrageEdit.setText("");
}
});
// 加载数据
viewModel.loadLiveData(liveId);
viewModel.connectWebSocket(liveId);
}
@Override
protected void onDestroy() {
super.onDestroy();
viewModel.disconnectWebSocket();
}
}
// LiveViewModel.java
public class LiveViewModel extends ViewModel {
private MutableLiveData
liveData = new MutableLiveData<>();
private MutableLiveData> barrages = new MutableLiveData<>();
private WebSocketClient webSocketClient;
public void loadLiveData(String liveId) {
// 调用API获取直播数据
LiveApi.getLiveDetail(liveId, new Callback() {
@Override
public void onResponse(Call call, Response response) {
if (response.isSuccessful()) {
liveData.postValue(response.body());
}
}
@Override
public void onFailure(Call call, Throwable t) {
// 错误处理
}
});
}
public void connectWebSocket(String liveId) {
// 创建WebSocket连接
webSocketClient = new WebSocketClient(URI.create("ws://your-server/live/ws")) {
@Override
public void onMessage(String message) {
// 处理WebSocket消息
JSONObject json = new JSONObject(message);
if ("barrage".equals(json.getString("type"))) {
Barrage barrage = new Gson().fromJson(json.getJSONObject("data").toString(), Barrage.class);
List current = barrages.getValue();
if (current == null) {
current = new ArrayList<>();
}
current.add(0, barrage);
barrages.postValue(current);
}
}
};
webSocketClient.connect();
}
public void sendBarrage(String liveId, String content) {
// 发送弹幕
JSONObject json = new JSONObject();
try {
json.put("live_id", liveId);
json.put("content", content);
json.put("color", "#FFFFFF");
json.put("position", 0);
if (webSocketClient != null && webSocketClient.isOpen()) {
webSocketClient.send(json.toString());
}
} catch (JSONException e) {
e.printStackTrace();
}
}
public void disconnectWebSocket() {
if (webSocketClient != null) {
webSocketClient.close();
}
}
// Getter方法...
}
```
## iOS实现 (Objective-C)
```objectivec
// LiveViewController.h
@interface LiveViewController : UIViewController
@property (nonatomic, strong) NSString *liveId;
@property (nonatomic, strong) AVPlayer *player;
@property (nonatomic, strong) AVPlayerLayer *playerLayer;
@property (nonatomic, strong) UITableView *barrageTableView;
@property (nonatomic, strong) UITextField *barrageTextField;
@property (nonatomic, strong) NSMutableArray *barrages;
@property (nonatomic, strong) SRWebSocket *webSocket;
@end
// LiveViewController.m
@implementation LiveViewController
- (void)viewDidLoad {
[super viewDidLoad];
// 初始化UI
[self setupUI];
// 加载数据
[self loadLiveData];
// 连接WebSocket
[self connectWebSocket];
}
- (void)setupUI {
// 视频播放器
self.player = [AVPlayer playerWithURL:[NSURL URLWithString:@""]];
self.playerLayer = [AVPlayerLayer playerLayerWithPlayer:self.player];
self.playerLayer.frame = CGRectMake(0, 0, self.view.bounds.size.width, self.view.bounds.size.height * 0.7);
[self.view.layer addSublayer:self.playerLayer];
// 弹幕列表
self.barrageTableView = [[UITableView alloc] initWithFrame:CGRectMake(0, self.view.bounds.size.height * 0.7, self.view.bounds.size.width, self.view.bounds.size.height * 0.2) style:UITableViewStylePlain];
self.barrageTableView.delegate = self;
self.barrageTableView.dataSource = self;
self.barrageTableView.backgroundColor = [UIColor clearColor];
self.barrageTableView.separatorStyle = UITableViewCellSeparatorStyleNone;
[self.view addSubview:self.barrageTableView];
// 弹幕输入框
UIView *inputView = [[UIView alloc] initWithFrame:CGRectMake(0, self.view.bounds.size.height * 0.9, self.view.bounds.size.width, 50)];
inputView.backgroundColor = [UIColor colorWithWhite:0 alpha:0.7];
self.barrageTextField = [[UITextField alloc] initWithFrame:CGRectMake(10, 10, self.view.bounds.size.width - 80, 30)];
self.barrageTextField.placeholder = @"发个弹幕吧~";
self.barrageTextField.textColor = [UIColor whiteColor];
self.barrageTextField.backgroundColor = [UIColor clearColor];
UIButton *sendButton = [UIButton buttonWithType:UIButtonTypeSystem];
sendButton.frame = CGRectMake(self.view.bounds.size.width - 70, 10, 60, 30);
[sendButton setTitle:@"发送" forState:UIControlStateNormal];
[sendButton setTitleColor:[UIColor whiteColor] forState:UIControlStateNormal];
[sendButton addTarget:self action:@selector(sendBarrage) forControlEvents:UIControlEventTouchUpInside];
[inputView addSubview:self.barrageTextField];
[inputView addSubview:sendButton];
[self.view addSubview:inputView];
}
- (void)loadLiveData {
NSString *url = [NSString stringWithFormat:@"%@", self.liveId];
[[AFHTTPSessionManager manager] GET:url parameters:nil progress:nil success:^(NSURLSessionDataTask * _Nonnull task, id _Nullable responseObject) {
NSDictionary *data = responseObject[@"data"];
NSString *streamUrl = data[@"stream_url"];
// 播放视频
self.player = [AVPlayer playerWithURL:[NSURL URLWithString:streamUrl]];
[self.player play];
} failure:^(NSURLSessionDataTask * _Nullable task, NSError * _Nonnull error) {
NSLog(@"Error: %@", error);
}];
}
- (void)connectWebSocket {
NSURL *url = [NSURL URLWithString:@"ws://your-server/live/ws"];
self.webSocket = [[SRWebSocket alloc] initWithURL:url];
self.webSocket.delegate = self;
[self.webSocket open];
}
- (void)sendBarrage {
NSString *content = self.barrageTextField.text;
if (content.length == 0) return;
NSDictionary *data = @{
@"live_id": self.liveId,
@"content": content,
@"color": @"#FFFFFF",
@"position": @0
};
NSError *error;
NSData *jsonData = [NSJSONSerialization dataWithJSONObject:data options:0 error:&error];
if (!error) {
[self.webSocket send:jsonData];
self.barrageTextField.text = @"";
}
}
#pragma mark - SRWebSocketDelegate
- (void)webSocket:(SRWebSocket *)webSocket didReceiveMessage:(id)message {
NSDictionary *dict = [NSJSONSerialization JSONObjectWithData:[message dataUsingEncoding:NSUTF8StringEncoding] options:0 error:nil];
if ([dict[@"type"] isEqualToString:@"barrage"]) {
Barrage *barrage = [[Barrage alloc] initWithDictionary:dict[@"data"] error:nil];
[self.barrages insertObject:barrage atIndex:0];
[self.barrageTableView reloadData];
}
}
#pragma mark - UITableViewDataSource
- (NSInteger)tableView:(UITableView *)tableView numberOfRowsInSection:(NSInteger)section {
return self.barrages.count;
}
- (UITableViewCell *)tableView:(UITableView *)tableView cellForRowAtIndexPath:(NSIndexPath *)indexPath {
UITableViewCell *cell = [tableView dequeueReusableCellWithIdentifier:@"BarrageCell"];
if (!cell) {
cell = [[UITableViewCell alloc] initWithStyle:UITableViewCellStyleDefault reuseIdentifier:@"BarrageCell"];
cell.backgroundColor = [UIColor clearColor];
cell.textLabel.textColor = [UIColor whiteColor];
}
Barrage *barrage = self.barrages[indexPath.row];
cell.textLabel.text = barrage.content;
return cell;
}
@end
```
## 实时通信方案
**WebSocket服务端实现 (Swoole)**
```objectivec
namespace app\websocket;
use think\swoole\Websocket;
class LiveWebsocket
{
protected $websocket;
// 用户连接
protected $connections = [];
public function __construct(Websocket $websocket)
{
$this->websocket = $websocket;
}
// 连接建立
public function onOpen($ws, $request)
{
$liveId = $request->get['live_id'];
$userId = $request->get['user_id'];
$this->connections[$liveId][$userId] = $ws->fd;
// 更新观看人数
$count = count($this->connections[$liveId] ?? []);
$this->websocket->emit('viewer_count', [
'live_id' => $liveId,
'count' => $count
]);
}
// 收到消息
public function onMessage($ws, $frame)
{
$data = json_decode($frame->data, true);
switch ($data['type']) {
case 'barrage':
// 广播弹幕
$this->broadcastToLive($data['data']['live_id'], [
'type' => 'barrage',
'data' => $data['data']
]);
break;
case 'heartbeat':
// 心跳检测
break;
}
}
// 连接关闭
public function onClose($ws, $fd)
{
foreach ($this->connections as $liveId => $users) {
if (($key = array_search($fd, $users)) !== false) {
unset($this->connections[$liveId][$key]);
// 更新观看人数
$count = count($this->connections[$liveId] ?? []);
$this->websocket->emit('viewer_count', [
'live_id' => $liveId,
'count' => $count
]);
break;
}
}
}
// 广播到指定直播间
protected function broadcastToLive($liveId, $data)
{
if (isset($this->connections[$liveId])) {
foreach ($this->connections[$liveId] as $fd) {
$this->websocket->push($fd, json_encode($data));
}
}
}
}
```
**这个实现方案涵盖了从后端到前端,再到移动端的完整实现,您可以根据实际需求进行调整和扩展。**
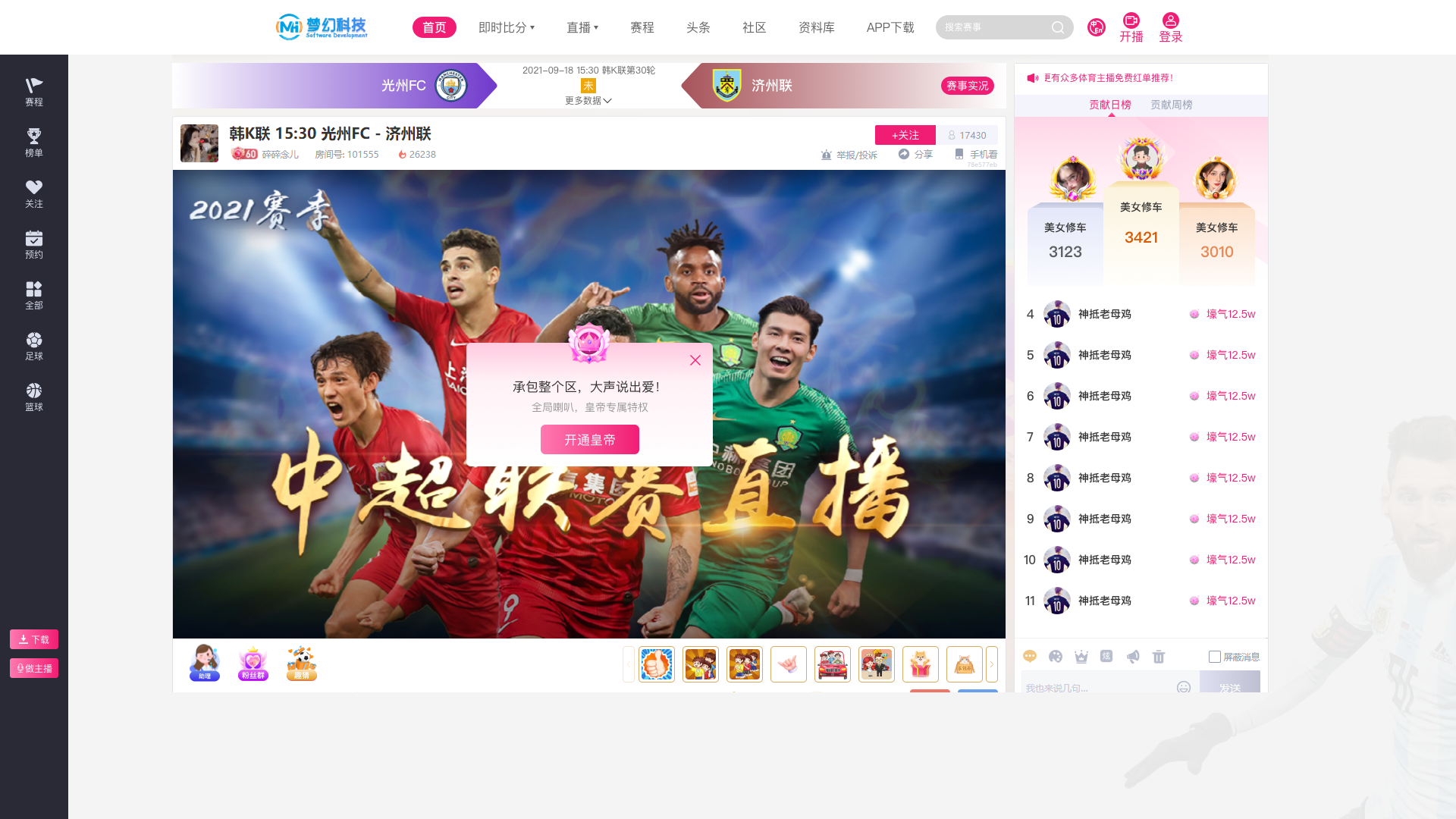