最近一直在找工作,复习了一下设计模式,现总结一下与Cuer一起Share一下:
一、 单例(Singleton)模式
单例模式的特点:
单例类只能有一个实例。
单例类必须自己创建自己的唯一实例。
单例类必须给所有其它对象提供这一实例。
单例模式应用:
每台计算机可以有若干个打印机,但只能有一个Printer Spooler,避免两个打印作业同时输出到打印机。
一个具有自动编号主键的表可以有多个用户同时使用,但数据库中只能有一个地方分配下一个主键编号。否则会出现主键重复。
二、 Singleton模式的结构:
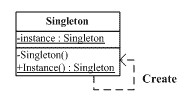
Singleton模式包含的角色只有一个,就是Singleton。Singleton拥有一个私有构造函数,确保用户无法通过new直接实例它。除此之外,该模式中包含一个静态私有成员变量instance与静态公有方法Instance()。Instance方法负责检验并实例化自己,然后存储在静态成员变量中,以确保只有一个实例被创建。(关于线程问题以及C#所特有的Singleton将在后面详细论述)。
三、 在什么情形下使用单例模式:
使用Singleton模式有一个必要条件:在一个系统要求一个类只有一个实例时才应当使用单例模式。反过来,如果一个类可以有几个实例共存,就不要使用单例模式。
注意:
不要使用单例模式存取全局变量。这违背了单例模式的用意,最好放到对应类的静态成员中。
不要将数据库连接做成单例,因为一个系统可能会与数据库有多个连接,并且在有连接池的情况下,应当尽可能及时释放连接。Singleton模式由于使用静态成员存储类实例,所以可能会造成资源无法及时释放,带来问题。
四.Singleton的代码实现
(1)C++版本
#include <iostream>
using namespace std;
class Singleton { public: static Singleton *Instance() { if (NULL == _instance) { _instance = new Singleton(); }
return _instance; } void method1(){cout << " method1 is done~ " << endl;};
protected: Singleton(void){ cout <<" Singleton() " << endl;}; virtual ~Singleton(){cout << "~singletont()" <<endl; }
static Singleton* _instance; };
//static 变量需要在类定义外面进行初始化
Singleton* Singleton::_instance = NULL;
int main() { cout << "Hello, world~!" << endl; Singleton::Instance()->method1(); Singleton::Instance()->method1(); return 0; }
|
(2) C#版本
sealed class Singleton { private Singleton(); public static readonly Singleton Instance = new Singleton(); }
|
//实现延迟初始化
public sealed class Singleton { Singleton() { } public static Singleton GetInstance() { return Nested.instance; } class Nested { // Explicit static constructor to tell C# compiler
// not to mark type as beforefieldinit
static Nested() { } internal static readonly Singleton instance = new Singleton(); } }
|
(3)JAVA版本
Public class Singleton{ private static Singleton uniqueInstance; private Singleton(){} public static synchronized Singleton getInstance(){ if(uniqueInstance == null){ uniqueInstance = new Singleton(); } return uniqueInstance; } // other useful methods here } /*这种写法可以实现单件模式,既简单又有效,利用了synchronized的同步方法。但是会带来严重的性能问题。同步一个方法可能造成程序执行效率下降100倍。因此,如果将getInstance()放到频繁运行的地方,就要重新考虑自己的设计了。 */
|
五参考资料
1.http://www.cppblog.com/SoRoMan/archive/2007/12/30/10140.html?opt=admin
2. http://leo-faith.javaeye.com/blog/177779
阅读(1784) | 评论(0) | 转发(0) |